Python and WebSockets 101: Real-time Communication
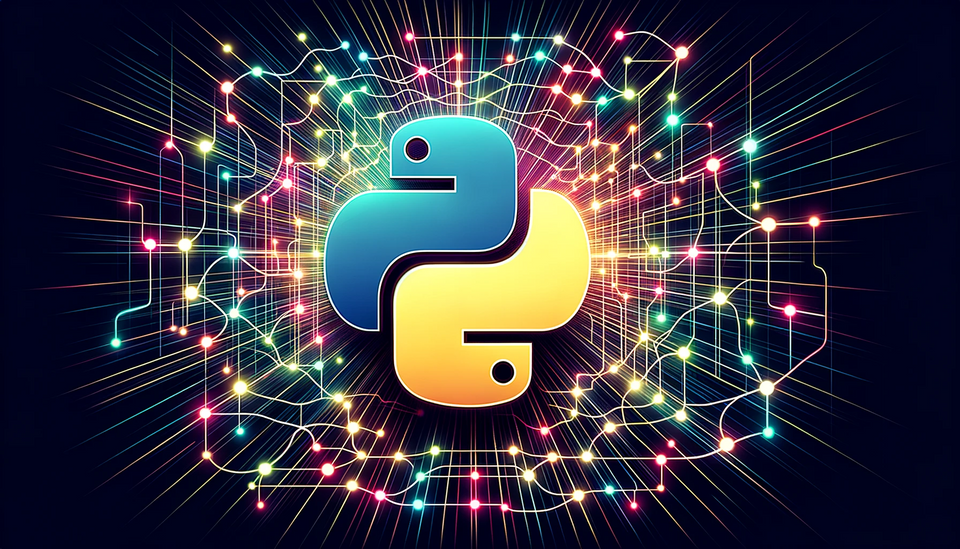
Introduction
WebSockets enable real-time, bi-directional communication between a client and a server over a single, long-lived connection. This article delves into how to work with WebSockets in Python, avoiding web frameworks to focus purely on the tech itself.
Table of Contents
- What are WebSockets?
- Python's
websockets
Library - Building a Simple WebSocket Server/Client
- Security Concerns
- Conclusion
What are WebSockets?
WebSockets are a communications protocol that provides a full-duplex communication channel over a single, long-lived TCP connection. The WebSocket Protocol was standardized by the IETF as RFC 6455 in 2011, and it has since gained widespread adoption for real-time, interactive applications where low latency and high interactivity are key requirements.
The WebSocket Handshake
The WebSocket connection starts with an HTTP handshake. During this phase, the client and server agree to upgrade the connection to a WebSocket connection. This is a one-time process and involves exchanging specific HTTP headers to indicate that both parties support WebSocket communication. Once the handshake is successful, the connection switches from HTTP to WebSocket, enabling a persistent, low-latency communication channel.
Full-duplex Communication
One of the most significant advantages of WebSockets is the full-duplex communication channel it establishes. In a full-duplex channel, both the client and server can send and receive messages simultaneously without closing the connection. This is different from HTTP, where each request-response cycle involves opening and closing a connection, leading to higher latency.
How is it Different from HTTP?
HTTP is a stateless protocol, which means that each request from a client to a server is independent and may not necessarily maintain any state information between subsequent requests. WebSockets, on the other hand, maintain state and allow for a persistent connection between the client and server. This makes WebSockets ideal for applications where real-time updates and rapid data exchange are essential, such as chat applications, online gaming, and financial trading platforms.
Python's websockets
Library
Python has a powerful library for working with WebSockets called websockets
, which allows you to manage WebSocket connections without the need for a web framework.
To install it, run:
pip install websockets
Building a Simple WebSocket Server/Client
Prerequisites
- Python 3.6 or higher
websockets
library installed (You can install it usingpip install websockets
)
Setting Up the WebSocket Server
You can build a simple WebSocket server using Python's websockets
and asyncio
libraries. Below is a sample code snippet:
# server.py
import asyncio
import websockets
async def echo(websocket, path):
async for message in websocket:
print(f"Someone said {message}")
await websocket.send(message)
start_server = websockets.serve(echo, 'localhost', 8765)
asyncio.get_event_loop().run_until_complete(start_server)
asyncio.get_event_loop().run_forever()
This code starts a WebSocket server on ws://localhost:8765/
.
Creating a WebSocket Client
You can also create a WebSocket client using the websockets
library. Here's how:
# client.py
import asyncio
import websockets
async def hello():
uri = "ws://localhost:8765"
async with websockets.connect(uri) as websocket:
message = input("Enter your message: ") # Get user input
await websocket.send(message) # Send the user's message to the WebSocket server
response = await websocket.recv() # Receive server response
print(f"Server Response: {response}") # Print the server response
asyncio.get_event_loop().run_until_complete(hello())
Run the Server: Open a terminal window, navigate to the directory where server.py
is located, and run python server.py
. Your WebSocket server will start at ws://localhost:8765/
.
Run the Client: Open another terminal window, navigate to the directory where client.py
is located, and run python client.py
.
Interact: Once the client is running, you'll be prompted to enter a message. Type your message and press Enter. You should see your message echoed back by the server as "Server Response: [Your Message]".
As you can try, not everyone connected to the server will receive the messages you send to the server, and this is we will see in a near future.
Such a setup offers an excellent opportunity for hands-on learning, especially in a course setting. Students tend to grasp the concept quickly, often finding it both enlightening and entertaining.
What makes this exercise particularly engaging is that it turns the classroom into a miniature real-time network, allowing students to immediately see the results of their code changes. The instant feedback loop not only makes the learning process more enjoyable but also fosters a sense of accomplishment.
It's not just about grasping the technicalities of WebSockets; it's also about witnessing the fun and immediate impact of real-time communication. This simple yet powerful exercise often becomes the highlight of the class, as students love to play around with it, sending messages, tweaking code, and exploring the possibilities of real-time data exchange.
Conclusion
In summary, WebSockets stand as a transformative technology that has significantly impacted the landscape of real-time communication in modern applications. Their ability to facilitate full-duplex, bi-directional interactions between clients and servers sets them apart from traditional HTTP communication methods, which are constrained by the limitations of request-response cycles.
Python's websockets
library further simplifies the implementation process, offering a clean and straightforward approach to setting up both WebSocket servers and clients. This ease of use, combined with Python's robust ecosystem, makes it an excellent choice for anyone looking to delve into real-time communication features in their applications.
However, the powerful capabilities of WebSockets come with their own set of challenges, particularly in the realm of security. It is essential to understand the associated risks, such as potential vulnerabilities to data interception if not using WSS (WebSocket Secure), and to implement proper security measures to mitigate these risks. Issues like authentication, encryption, and rate-limiting should be high on the checklist of any developer working with WebSockets.
As we move towards an increasingly interconnected digital world, the importance of real-time communication can only be expected to grow. WebSockets, with their low latency and high efficiency, are likely to remain at the forefront of this evolution. Understanding the technology and how to implement it securely will, therefore, be invaluable skills for developers and IT professionals alike.